Mastering Error Handling in Nuxt: A Comprehensive Guide
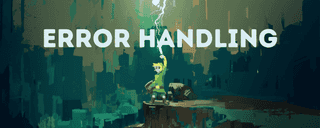
Outline
In any web application, error handling is a critical aspect that ensures a smooth user experience and robust system behavior. Nuxt, a full-stack framework based on Vue.js, offers a sophisticated error-handling mechanism that allows developers to gracefully manage errors across different contexts, whether during server-side rendering (SSR), client-side rendering (CSR), or within the Nitro server.
In this blog, we'll explore how to catch and handle errors in Nuxt, covering various scenarios from Vue rendering lifecycle errors to handling chunk loading failures. By the end, you'll have a solid understanding of how to implement effective error handling strategies in your Nuxt applications.
Understanding the Sources of Errors in Nuxt
Before diving into error handling techniques, it's essential to understand where errors can originate in a Nuxt application. Being a full-stack framework, Nuxt applications can encounter errors in several contexts:
- Vue Rendering Lifecycle Errors (SSR & CSR): These errors occur during the rendering process of Vue components, both on the server and client sides.
- Startup Errors (SSR & CSR): Errors can occur during the startup process of a Nuxt application, including the execution of plugins, the creation of the app, and its mounting.
- Nitro Server Lifecycle Errors: These errors occur within the Nitro server, typically when processing server routes or rendering the application into HTML.
- JS Chunk Loading Errors: Errors can happen when JavaScript chunks fail to load, often due to network issues or mismatches between the client and server after a new deployment.
Handling Vue Errors
Vue errors are among the most common in Nuxt applications. Fortunately, Nuxt provides several hooks and tools to capture and manage these errors effectively.
Using onErrorCaptured
Vue's onErrorCaptured
lifecycle hook allows you to capture errors in child components and prevent them from propagating upwards. This can be useful for isolating errors and handling them at a component level.
Global Error Handling with vue:error
Nuxt extends Vue's capabilities with a vue:error
hook, which catches errors that propagate to the top level. This hook is particularly useful when you need to handle errors globally, such as reporting them to an external error-tracking service.
Here’s an example of how to implement a global error handler in Nuxt:
// plugins/error-handler.ts
export default defineNuxtPlugin((nuxtApp) => {
nuxtApp.vueApp.config.errorHandler = (error, instance, info) => {
// Handle the error, e.g., report it to a service
}
nuxtApp.hook('vue:error', (error, instance, info) => {
// Handle the error, e.g., report it to a service
})
})
This code snippet sets up a global error handler that captures all Vue errors, allowing you to handle them consistently across your application.
Managing Startup Errors
Startup errors can occur during the initialization phase of your Nuxt application, affecting both SSR and CSR. Nuxt provides the app:error
hook to capture these errors.
Common Sources of Startup Errors
Startup errors may arise from:
- Running Nuxt plugins
- Processing
app:created
andapp:beforeMount
hooks - Rendering your Vue app into HTML (during SSR)
- Mounting the app on the client side
You can handle these errors by hooking into app:error
, ensuring that your application can recover gracefully from initialization failures.
Handling Nitro Server Errors
Nitro, the server engine behind Nuxt, can encounter errors during server-side processes. Although you cannot define a server-side handler for these errors directly, you can still manage them by rendering a custom error page.
Dealing with JS Chunk Loading Errors
JS chunk loading errors can disrupt the client-side experience, especially when a network issue or a new deployment invalidates old chunk URLs. Nuxt provides built-in support for handling these errors by performing a hard reload when a chunk fails to load.
Customizing Chunk Loading Error Handling
You can modify this behavior by setting experimental.emitRouteChunkError
in your configuration:
export default defineNuxtConfig({
experimental: {
emitRouteChunkError: 'manual' // Set to 'false' to disable, or 'manual' to handle yourself
}
})
If you choose to handle these errors manually, consider implementing a custom retry mechanism or displaying a user-friendly message.
Rendering Custom Error Pages
When Nuxt encounters a fatal error, it renders an error page. You can customize this page by adding an error.vue
file to your project. This file allows you to define how errors should be displayed to users.
<!-- error.vue -->
<script setup lang="ts">
import type { NuxtError } from '#app'
const props = defineProps({
error: Object as () => NuxtError
})
const handleError = () => clearError({ redirect: '/' })
</script>
<template>
<div>
<h2>{{ error.statusCode }}</h2>
<button @click="handleError">Clear errors</button>
</div>
</template>
In this example, the error page displays the status code and provides a button to clear the error, optionally redirecting the user to a "safe" page.
Using the useError
Composable
Nuxt offers the useError
composable to access the global error being handled. This utility is particularly useful in middleware or components where you need to check the current error state.
const error = useError()
if (error.value) {
// Handle the error
}
Creating and Showing Errors
Nuxt provides utilities like createError
, showError
, and clearError
to manage errors programmatically.
createError
: Generates an error object with additional metadata, useful for both server-side and client-side error handling.showError
: Triggers a full-screen error page from anywhere in your application.clearError
: Clears the current error and optionally redirects the user.
These utilities allow you to manage errors flexibly, whether you need to trigger a custom error page or handle errors in specific components.
Error Boundaries with <NuxtErrorBoundary>
Nuxt also provides a <NuxtErrorBoundary>
component to handle client-side errors without disrupting the entire application. This component is especially useful when you want to catch errors locally within a specific part of your app.
<template>
<NuxtErrorBoundary @error="someErrorLogger">
<!-- Default slot for your content -->
<template #error="{ error, clearError }">
<p>An error occurred: {{ error.message }}</p>
<button @click="clearError">Clear Error</button>
</template>
</NuxtErrorBoundary>
</template>
This setup ensures that errors in the component's slot don't affect the rest of the application, providing a more resilient user experience.
Conclusion
Error handling in Nuxt is a comprehensive system that spans across client-side rendering, server-side processes, and even network-related issues like JS chunk loading failures. By leveraging Nuxt's hooks, utilities, and components, you can create a robust error-handling strategy that not only ensures a smooth user experience but also helps you maintain a healthy and maintainable codebase.
Whether you're building small applications or large-scale systems, mastering error handling in Nuxt will empower you to manage unexpected issues effectively, keeping your application resilient and your users happy.
Let's Connect
Youtube •GitHub •LinkedIn