How to Do OnPageSEO on Nuxt APP
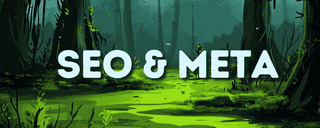
Outline
Nuxt.js is a powerful framework built on Vue.js, designed for building high-performance web applications. It not only simplifies development but also provides out-of-the-box tools to help developers optimize for Search Engine Optimization (SEO). In this blog, we’ll dive into SEO and meta tags in Nuxt.js, explore the core techniques to optimize your app for search engines, and share tips, tricks, and optimization methods that can enhance your app’s overall experience.
What Is SEO and Why Does It Matter?
Search Engine Optimization (SEO) is the process of improving a website’s visibility on search engines like Google, Bing, or Yahoo. Good SEO practices ensure that your content is easily found and ranked higher by search engine crawlers. This results in increased organic traffic to your site, higher engagement, and better user retention.
Nuxt.js is primarily focused on server-side rendering (SSR) and static site generation (SSG), both of which are advantageous for SEO. SSR ensures that search engine crawlers receive pre-rendered HTML content, making it easier for them to index your pages. On the other hand, SSG can generate fully static HTML files, allowing for faster page loads, a key factor for SEO.
Meta tags play a crucial role in SEO because they provide essential information to search engines and social media platforms. These tags can influence how your website appears in search results, improve click-through rates, and control how pages are shared on social media.
Meta Tags in Nuxt.js
Nuxt.js offers several built-in ways to manage meta tags. The framework provides robust support for adding and updating meta information in your pages dynamically using head()
within components or pages.
Adding Meta Tags in Nuxt.js
Here’s how you can add meta tags in a Nuxt.js page using the head()
method.
export default {
head() {
return {
title: 'My Nuxt App',
meta: [
{ hid: 'description', name: 'description', content: 'This is a sample Nuxt.js application' },
{ hid: 'keywords', name: 'keywords', content: 'nuxt, vue, seo, meta tags' },
{ hid: 'og:title', property: 'og:title', content: 'My Nuxt App' },
{ hid: 'og:description', property: 'og:description', content: 'This is a sample Nuxt.js application' },
{ hid: 'og:image', property: 'og:image', content: '/my-app-image.png' },
]
}
}
}
hid
: Unique identifier to avoid duplicate meta tags.name
orproperty
: Defines what the meta tag represents.content
: The actual value of the meta tag.
Dynamic Meta Tags for Better SEO
Nuxt.js allows you to dynamically set meta tags, which is crucial for optimizing pages with dynamic content (like blogs or product listings). You can fetch data from an API or a store like Pinia and use it to populate your meta tags.
export default {
async asyncData({ params }) {
const post = await fetchPost(params.id);
return { post };
},
head() {
return {
title: this.post.title,
meta: [
{ hid: 'description', name: 'description', content: this.post.excerpt },
{ hid: 'og:title', property: 'og:title', content: this.post.title },
{ hid: 'og:description', property: 'og:description', content: this.post.excerpt },
{ hid: 'og:image', property: 'og:image', content: this.post.image },
]
};
}
}
Nuxt.js SEO Tricks and Best Practices
Now that you understand how to use meta tags in Nuxt.js, let’s explore some advanced SEO tips and optimization strategies to improve your app's experience.
1. Enable SSR or Static Site Generation
Nuxt.js supports both Server-Side Rendering (SSR) and Static Site Generation (SSG). Both approaches are beneficial for SEO because they deliver pre-rendered content to search engine crawlers. You can enable these modes through the nuxt.config.js
file.
- SSR Mode:
export default {
ssr: true,
};
- SSG Mode:
export default {
target: 'static',
};
2. Use nuxt-seo
Module
For streamlined SEO management, consider using the nuxt-seo module. This plugin allows for easier configuration of SEO properties and supports features like canonical URLs, robots.txt, and automatic generation of Open Graph and Twitter card tags.
Install the module:
npm install nuxt-seo
Add it to nuxt.config.js
:
export default {
modules: [
'nuxt-seo',
],
seo: {
baseUrl: 'https://mywebsite.com',
name: 'My Nuxt App',
titleTemplate: '%name% - %title%',
description: 'This is a fantastic Nuxt.js application!',
keywords: ['nuxt', 'seo', 'meta tags'],
openGraph: {
type: 'website',
siteName: 'My Nuxt App',
title: 'My Nuxt App',
description: 'This is a fantastic Nuxt.js application!',
url: 'https://mywebsite.com',
image: '/my-app-image.png',
},
}
}
3. Optimize for Mobile SEO
A significant portion of traffic comes from mobile devices, and Google prioritizes mobile-first indexing. Ensure your site is responsive and mobile-friendly by following these steps:
- Use responsive meta tags:
<meta name="viewport" content="width=device-width, initial-scale=1">
- Make sure your CSS uses flexible layouts like Flexbox and Grid to ensure responsiveness.
4. Preload Critical Resources
For faster page loads, especially on mobile, use the preload tag for critical resources like fonts, CSS, or JavaScript. Preloading hints help the browser prioritize fetching these assets.
In nuxt.config.js
:
export default {
head: {
link: [
{
rel: 'preload',
href: '/fonts/my-font.woff2',
as: 'font',
type: 'font/woff2',
crossorigin: 'anonymous'
}
]
}
}
5. Image Optimization
Large images can slow down your website, impacting both user experience and SEO rankings. Use these techniques:
- Compress images before serving them.
- Use
nuxt-image
for dynamic image optimization:
npm install @nuxt/image
In nuxt.config.js
:
export default {
modules: ['@nuxt/image'],
image: {
domains: ['cdn.example.com'],
presets: {
thumbnail: { modifiers: { width: 300, height: 300 } }
}
}
}
6. Structured Data with JSON-LD
Google recommends using JSON-LD to add structured data to web pages. This helps search engines better understand your content.
In head()
:
export default {
head() {
return {
script: [
{
type: 'application/ld+json',
json: {
"@context": "http://schema.org",
"@type": "WebSite",
"name": "My Nuxt App",
"url": "https://mywebsite.com"
}
}
]
};
}
}
7. Lazy Load Images and Components
Lazy loading is an effective way to boost performance by loading only the content visible on the screen. This can improve page load times and the overall user experience, which indirectly benefits SEO.
You can enable lazy loading for images using Nuxt’s nuxt-img
component.
<nuxt-img src="/image.jpg" lazy />
For components, leverage dynamic imports
:
const LazyComponent = () => import('@/components/LazyComponent.vue');
Conclusion
Optimizing SEO and meta tags in Nuxt.js is essential for boosting your app’s discoverability and enhancing the user experience. By following the above tips, from configuring SSR/SSG to optimizing images and leveraging structured data, you can significantly improve your app’s performance in search rankings.
Take advantage of the Nuxt.js framework’s flexibility to fine-tune meta tags, optimize for mobile, and ensure your website is indexed correctly by search engines. With thoughtful planning and implementation, you can ensure a highly optimized and SEO-friendly web app.
Let's Connect
Youtube •GitHub •LinkedIn