Optimizing SEO in Your Nuxt App with Prerendering
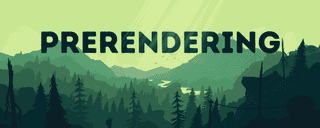
Outline
Search Engine Optimization (SEO) is a critical aspect of any web application, as it directly impacts visibility, user engagement, and overall success. If you’re using Nuxt, a powerful Vue.js framework, you’re in luck—Nuxt offers a suite of tools and features that make managing SEO and meta tags straightforward and highly customizable. In this blog, we’ll dive deep into how you can leverage Nuxt’s prerendering capabilities, reactive head management, and SEO enhancements to boost your app’s performance and visibility.
Nuxt's Prerendering and SEO Defaults
Nuxt is designed with performance and SEO in mind. Out-of-the-box, it provides sensible defaults that cater to most common use cases, which can be further customized based on your app’s specific needs. The central configuration file, nuxt.config.ts
, allows you to define global settings for your app’s <head>
section. For example:
export default defineNuxtConfig({
app: {
head: {
charset: 'utf-8',
viewport: 'width=device-width, initial-scale=1',
}
}
})
This basic setup ensures that your app uses a universal character set and responsive viewport settings, both essential for good SEO. However, this method doesn’t allow for reactive data—meaning you can`t dynamically change these settings based on user interactions or route changes. For more dynamic control, Nuxt provides composables like useHead and useSeoMeta, which we’ll explore next.
Programmatic and Reactive Head Management with useHead
For more granular control over your app's <head>
tags, the useHead
composable is a powerful tool. It allows you to manage meta tags and other head elements programmatically, making them reactive to changes in your app’s state.
Here’s an example of how to use useHead
in app.vue
:
<script setup lang="ts">
useHead({
title: 'My App',
meta: [
{ name: 'description', content: 'My amazing site.' }
],
bodyAttrs: {
class: 'test'
},
script: [
{ innerHTML: 'console.log(\'Hello world\')' }
]
})
</script>
This setup lets you dynamically control the page title, meta descriptions, and even inject scripts into the body. Since useHead is reactive, these properties can change as your app state or route changes, ensuring that search engines and users see the most relevant information at all times.
Simplified SEO Management with useSeoMeta
While useHead
offers comprehensive control, the useSeoMeta composable focuses specifically on SEO-related meta tags, simplifying the process further. It provides TypeScript support and enforces best practices by preventing common mistakes like misusing name
instead of property
. Here’s how you can use it:
<script setup lang="ts">
useSeoMeta({
title: 'My Amazing Site',
ogTitle: 'My Amazing Site',
description: 'This is my amazing site, let me tell you all about it.',
ogDescription: 'This is my amazing site, let me tell you all about it.',
ogImage: 'https://example.com/image.png',
twitterCard: 'summary_large_image',
})
</script>
With useSeoMeta
, you can define Open Graph tags, Twitter cards, and other SEO essentials in a flat, easy-to-manage object. This ensures consistency and accuracy across your app’s pages, which is crucial for effective SEO.
Dynamic Titles with titleTemplate
One of the most common needs in web development is setting dynamic page titles based on the current route or state. Nuxt’s titleTemplate
option allows you to create customizable title structures that can automatically append your site’s name to each page title:
<script setup lang="ts">
useHead({
titleTemplate: (titleChunk) => {
return titleChunk ? `${titleChunk} - Site Title` : 'Site Title';
}
})
</script>
By using a function for titleTemplate
, you gain full control over how titles are generated, enabling you to create a more personalized and engaging user experience.
Working with Nuxt's Head Components
In addition to composables, Nuxt provides a set of <Head>
, <Meta>
, <Link>
, and other components that allow you to directly interact with the document’s head section within your component templates. This approach is useful when you need to bind reactive data directly to head elements:
<template>
<div>
<Head>
<Title>{{ title }}</Title>
<Meta name="description" :content="title" />
<Style type="text/css" children="body { background-color: green; }" ></Style>
</Head>
<h1>{{ title }}</h1>
</div>
</template>
<script setup lang="ts">
const title = ref('Hello World')
</script>
This method ensures that your head tags are tightly integrated with the rest of your Vue components, making them more maintainable and easier to understand.
Advanced Features: Body Tags and External CSS
Nuxt also allows you to control where certain tags are inserted into the document. For instance, you can use the tagPosition:
'bodyClose'
option to append scripts or styles to the end of the <body>
tag, ensuring they load after the main content:
<script setup lang="ts">
useHead({
script: [
{
src: 'https://third-party-script.com',
tagPosition: 'bodyClose'
}
]
})
</script>
Additionally, integrating external CSS, such as Google Fonts, is straightforward with Nuxt’s head management tools:
<script setup lang="ts">
useHead({
link: [
{
rel: 'preconnect',
href: 'https://fonts.googleapis.com'
},
{
rel: 'stylesheet',
href: 'https://fonts.googleapis.com/css2?family=Roboto&display=swap',
crossorigin: ''
}
]
})
</script>
This ensures that your external resources are loaded efficiently and correctly, improving both performance and SEO.
Conclusion
Optimizing your Nuxt app for SEO doesn’t have to be complex. With Nuxt’s built-in tools like useHead
, useSeoMeta
, and various head components, you can easily manage and customize your app’s metadata, ensuring it’s always optimized for search engines and user experience. Whether you need reactive head management, dynamic titles, or advanced tag positioning, Nuxt has you covered. Dive into these features, experiment with your configurations, and watch your app’s SEO performance soar.
Let's Connect
Youtube •GitHub •LinkedIn